Using environment variables in Swift
在 Swift 中使用环境变量
Explore the importance of environment variables in Swift and how to use them.
探索环境变量在 Swift 中的重要性以及如何使用它们。
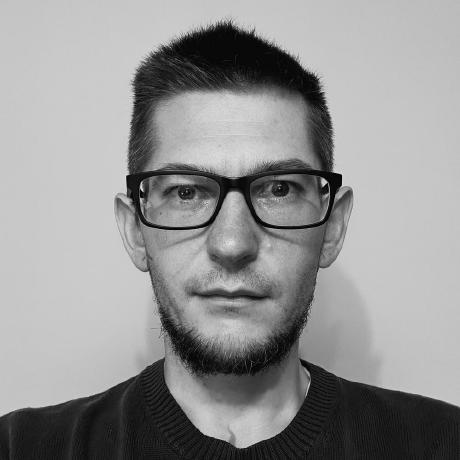
Environment variables are key-value pairs that can be used to alter the behavior of an application. The variables are part of the environment in which a process runs. The environment is injected during the runtime of the application. Environment variables can be set at the system level or they can be defined by the end-user.
环境变量是可用于更改应用程序行为的键值对。变量是进程运行环境的一部分。环境是在应用程序运行时注入的。环境变量可以在系统级别设置,也可以由最终用户定义。
Environment variables are commonly used for configuration purposes. It is possible to set different configuration values for development, testing and production environment. It is possible to use env vars as feature flags or to specify secrets and keys and keep them separate from the application codebase.
环境变量通常用于配置目的。可以为开发、测试和生产环境设置不同的配置值。可以使用环境变量作为功能标志或指定秘密和密钥并将它们与应用程序代码库分开。
For example, an app could take advantage of the LOG_LEVEL=trace
environment variable to set the log level using the Logging library in Swift. By using an env variable developers can get more detailed logs for debugging purposes and less verbose logs for production without changing the source code of the application itself.
例如,应用程序可以利用 LOG_LEVEL=trace
环境变量来使用 Swift 中的日志库设置日志级别。通过使用环境变量,开发人员可以获得更详细的日志以用于调试目的,并获得更简洁的日志以用于生产,而无需更改应用程序本身的源代码。
How to access environment variables in Swift?
如何在 Swift 中访问环境变量?
In Swift, it is possible to access environment variables using the ProcessInfo class. The ProcessInfo class is part of the Foundation framework.
在 Swift 中,可以使用 ProcessInfo 类访问环境变量。 ProcessInfo 类是 Foundation 框架的一部分。
Here's a quick example how to get the value of the LOG_LEVEL
variable:
以下是如何获取 LOG_LEVEL
变量值的简单示例:
import Foundation
let env = ProcessInfo.processInfo.environment
let value = env["LOG_LEVEL"] ?? "trace"
print(value)
The process info's environment is represented a [String: String]
dictionary. When requesting a specific key, the value is going to be an optional String
type.
进程信息的环境由 [String: String]
字典表示。当请求特定键时,该值将是可选的 String
类型。
The output of this script is trace, if the LOG_LEVEL
is not provided explicitly.
如果未显式提供 LOG_LEVEL
,则此脚本的输出为跟踪。
How to set environment variables?
如何设置环境变量?
There are several methods for configuring environment variables, and the approach you take depends on the specific tools you're working with.
配置环境变量的方法有多种,您所采用的方法取决于您正在使用的特定工具。
Here are some typical scenarios for setting up custom environment variables.
以下是设置自定义环境变量的一些典型场景。
Setting env vars using the command line
使用命令行设置环境变量
List the desired environment variables before the command when running a Swift package using a terminal window. Key-value pairs should be listed using the key=value
format, separated by a single space character.
使用终端窗口运行 Swift 包时,在命令之前列出所需的环境变量。键值对应使用 key=value
格式列出,并用单个空格字符分隔。
Here's how to define an explicit log level by using the swift run
command:
以下是如何使用 swift run
命令定义显式日志级别:
LOG_LEVEL=debug swift run
// output: "debug"
The command above makes the LOG_LEVEL
env variable set to trace
for the swift run
process. Traditionally environment variables are uppercased.
上面的命令将 swift run
进程的 LOG_LEVEL
环境变量设置为 trace
。传统上环境变量是大写的。
The export command extends the availability of an environment variable throughout the shell's entire lifecycle. Here's how to use it:
导出命令将环境变量的可用性扩展到 shell 的整个生命周期。使用方法如下:
echo $LOG_LEVEL // output: ""
export LOG_LEVEL=info
echo $LOG_LEVEL // output: "info"
swift run
// output: "info"
The echo
command is used to display variables in a shell script. Use the $ prefix and the name of the variable to access the value of it.
echo
命令用于显示 shell 脚本中的变量。使用 $ 前缀和变量名称来访问它的值。
Setting env vars using Xcode
使用 Xcode 设置环境变量
In Xcode, you can configure environment variables within the Scheme settings. The interface permits enabling or disabling specific key-value pairs for a particular run.
在 Xcode 中,您可以在方案设置中配置环境变量。该接口允许启用或禁用特定运行的特定键值对。
Here's how to reach the settings:
访问设置的方法如下:
- Open the project in Xcode.
在 Xcode 中打开项目。
- Select the "Product" > "Scheme" > "Edit Scheme..." menu item.
选择“产品”>“方案”>“编辑方案...”菜单项。
Alternatively, click on the Scheme name and select the "Edit Scheme..." menu item:
或者,单击方案名称并选择“编辑方案...”菜单项:
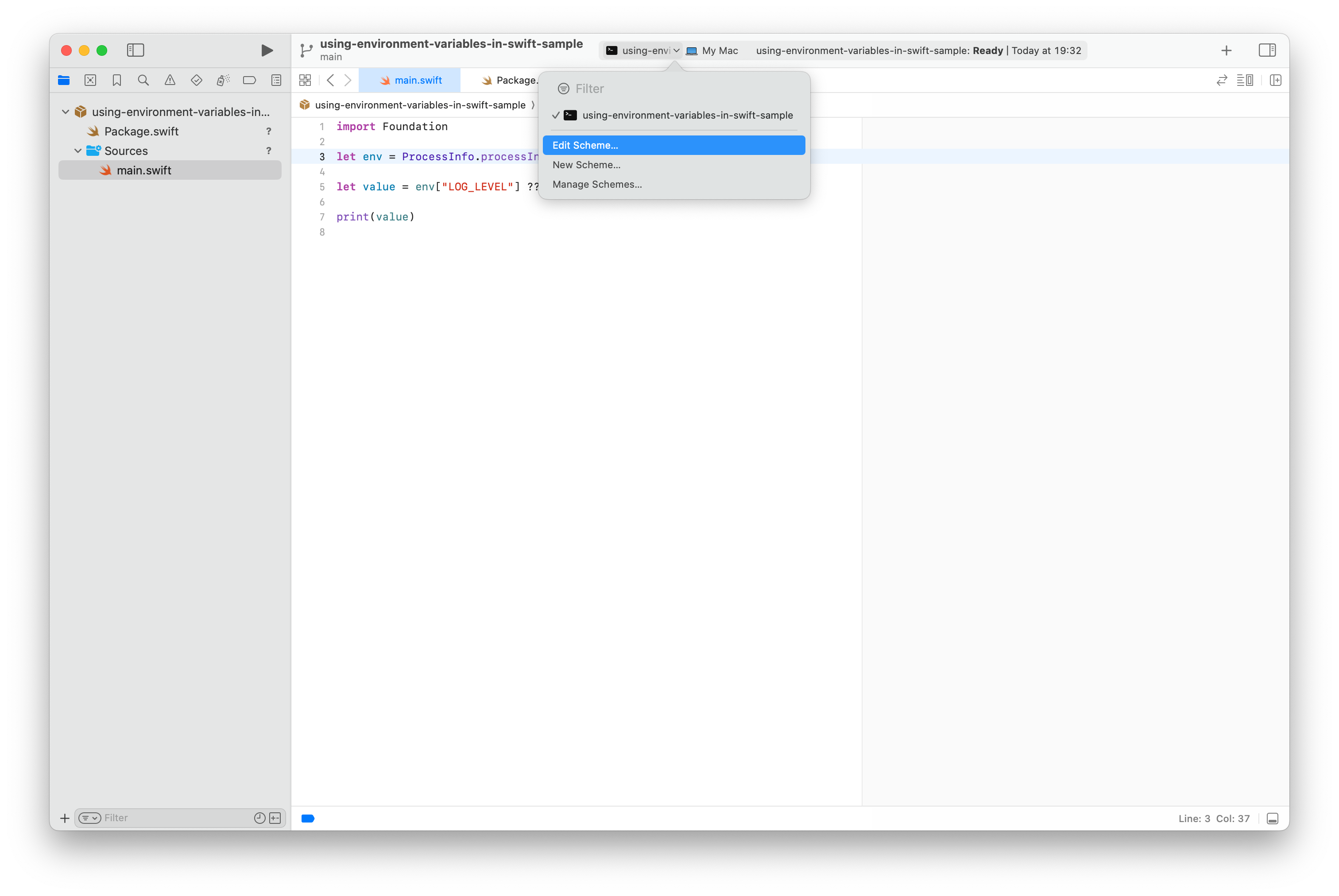
Inside the popup window: 在弹出窗口内:
- Select the "Run" option on the sidebar.
选择侧边栏上的“运行”选项。
- Select the "Arguments" tab.
选择“参数”选项卡。
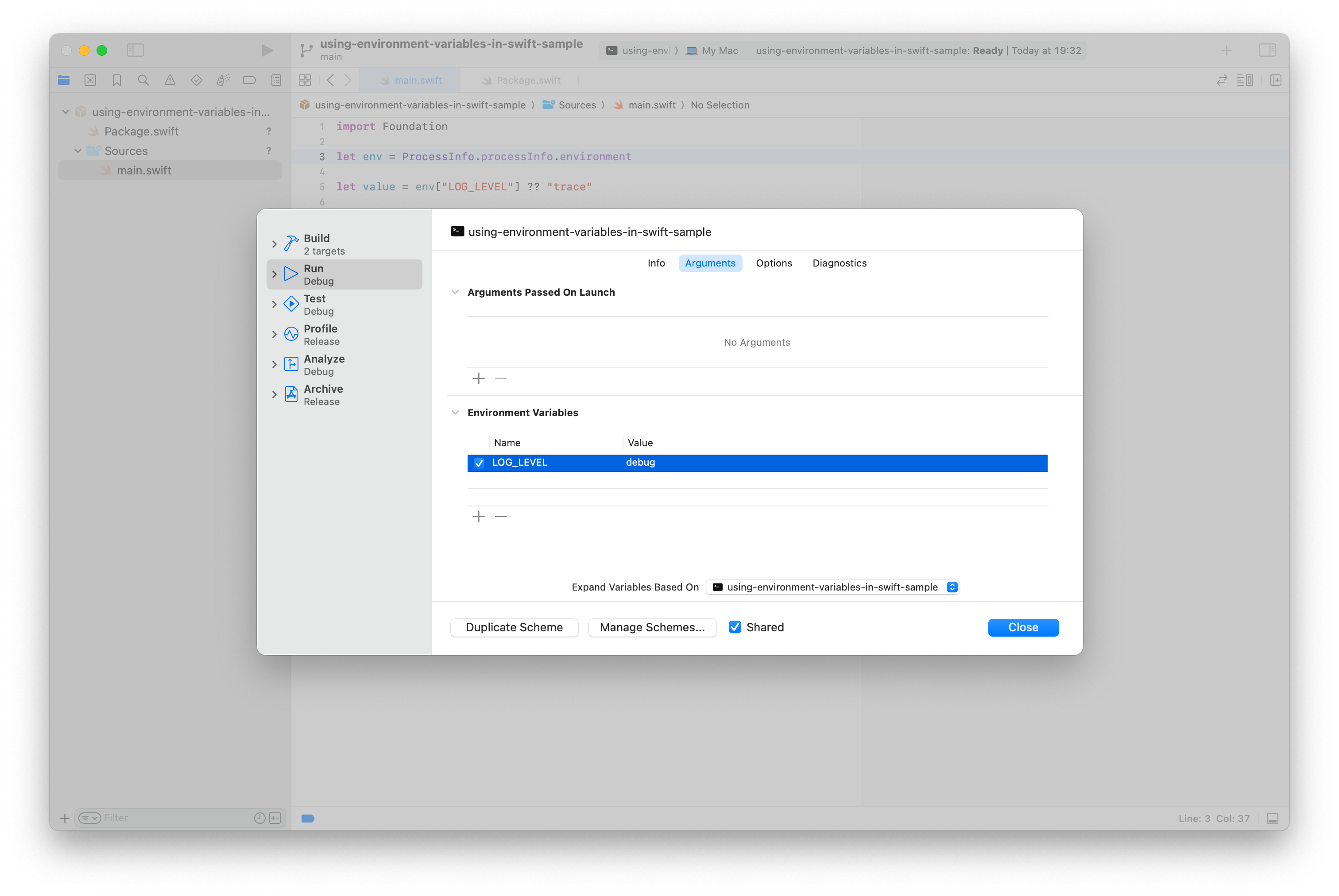
Finally, add a new entry into the "Environment Variables" section.
最后,在“环境变量”部分添加一个新条目。
Click "Close" to get back to the project and press the "Play" icon to run the app.
单击“关闭”返回项目,然后按“播放”图标运行应用程序。
Setting env vars using Visual Studio Code
使用 Visual Studio Code 设置环境变量
It is possible to develop Swift projects with VSCode using the official Swift extension.
可以使用官方 Swift 扩展通过 VSCode 开发 Swift 项目。
In order to set environment variables in the editor, open the .vscode/launch.json
file in your workspace or select the "Debug" > "Open Configurations" menu item.
为了在编辑器中设置环境变量,请在工作区中打开 .vscode/launch.json
文件或选择“调试”>“打开配置”菜单项。
Inside the launch configuration file simply add to a new env
property, if it's not present, with the desired key-value pairs:
在启动配置文件中,只需添加一个新的 env
属性(如果不存在)以及所需的键值对:
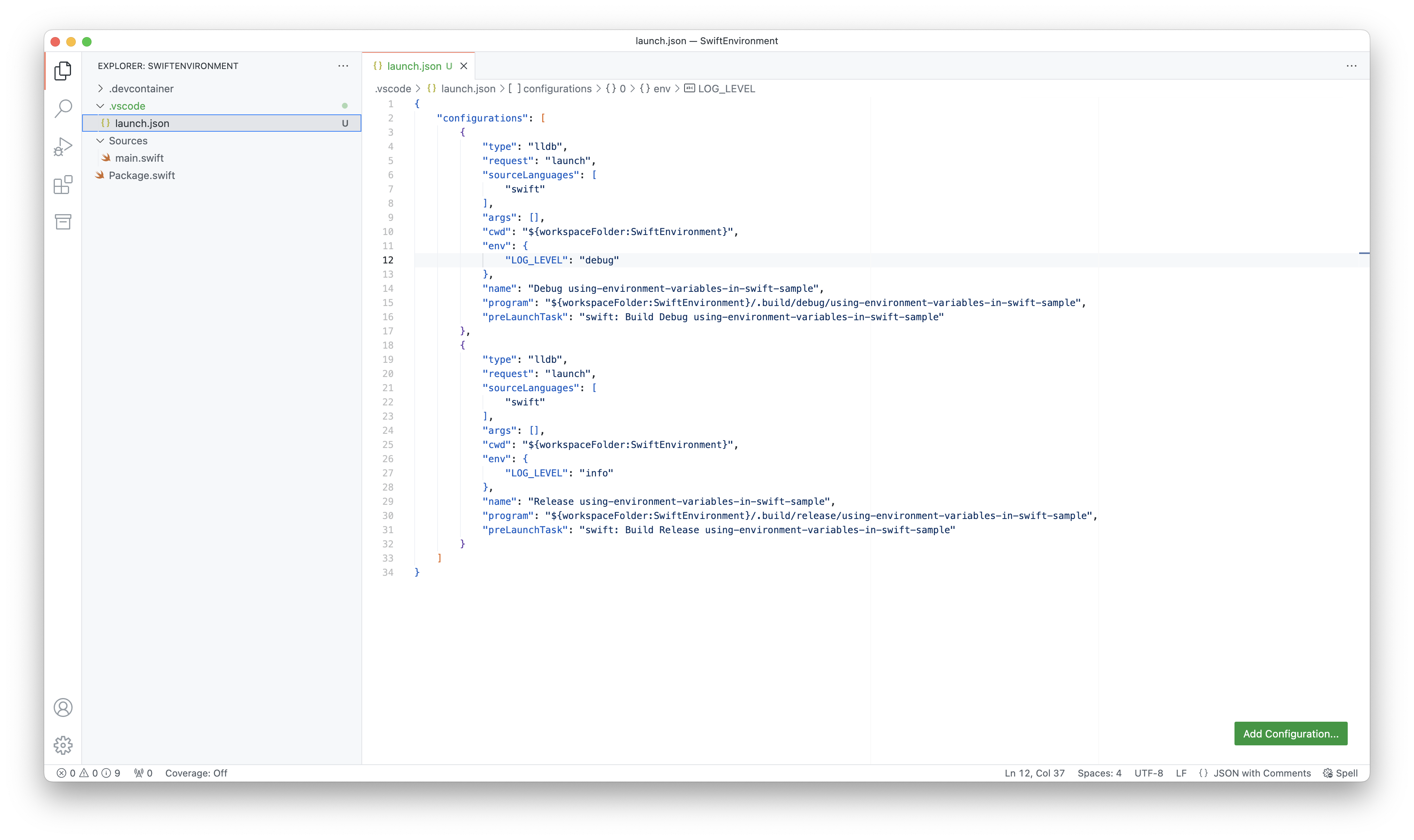
Save the launch config and run the project using the "Play" icon inside the "Run and Debug" panel or using the "Run" > "Start Debugging" menu item.
保存启动配置并使用“运行和调试”面板内的“播放”图标或使用“运行”>“开始调试”菜单项运行项目。
Using dotenv files 使用 dotenv 文件
A .env
file is a text file commonly used to store environment variables for a project. It contains key-value pairs in the form of KEY=VALUE
, where each line represents a different variable. Developers use libraries or tools to load the variables from the .env file into the application's environment. This allows the application to access these variables as if they were set directly in the system's environment.
.env
文件是一个文本文件,通常用于存储项目的环境变量。它包含 KEY=VALUE
形式的键值对,其中每一行代表一个不同的变量。开发人员使用库或工具将 .env 文件中的变量加载到应用程序的环境中。这允许应用程序访问这些变量,就像它们是直接在系统环境中设置的一样。
NOTE: The .env files should be excluded from git using .gitignore.
注意:应使用 .gitignore 将 .env 文件从 git 中排除。
The .env file provides a flexible and convenient way to manage environment-specific configuration settings in a project while keeping sensitive information secure and separate from the codebase.
.env 文件提供了一种灵活便捷的方法来管理项目中特定于环境的配置设置,同时保持敏感信息的安全并与代码库分离。
Various open-source Swift libraries exist for server-side application developers, providing functionality to parse dotenv files. For instance:
为服务器端应用程序开发人员提供了各种开源 Swift 库,提供解析 dotenv 文件的功能。例如:
Most of the modern web frameworks, have excellent support for loading dotenv files. Both Vapor and Hummingbird have a built-in solution to load and parse environment variables using these files.
大多数现代 Web 框架都对加载 dotenv 文件提供了出色的支持。 Vapor 和 Hummingbird 都有一个内置的解决方案来使用这些文件加载和解析环境变量。
Using the environment in Vapor
使用Vapor环境
Vapor's Environment API enables dynamic configuration of the application:
Vapor 的环境 API 支持应用程序的动态配置:
import Vapor
// configures your application
public func configure(_ app: Application) async throws {
// 1.
var logger = Logger(label: "vapor-logger")
logger.logLevel = .trace
// 2.
let logLevel = Environment.get("LOG_LEVEL")
// 3.
if let logLevel, let logLevel = Logger.Level(rawValue: logLevel) {
// 4.
logger.logLevel = logLevel
}
try routes(app)
}
- Set up a new Logger instance, set the default log level to .trace
设置一个新的Logger实例,将默认日志级别设置为.trace - Get the the raw log level as an optional String using the Environment
使用环境获取原始日志级别作为可选字符串 - Cast the log level to a
Logger.Level
enum, if it's a valid input
如果输入有效,则将日志级别转换为Logger.Level
枚举 - Set the log level based on the environment
根据环境设置日志级别
Vapor will look for dotenv files in the current working directory. If you're using Xcode, make sure to set the working directory by editing the scheme.
Vapor 将在当前工作目录中查找 dotenv 文件。如果您使用的是 Xcode,请确保通过编辑方案来设置工作目录。
Using the environment in Hummingbird 2
使用 Hummingbird 2 中的环境
In Hummingbird, it is possible to use the shared environment or load dotenv files using the static dotEnv()
method on the HBEnvironment struct:
在 Hummingbird 中,可以使用共享环境或使用 HBEnvironment 结构上的静态 dotEnv()
方法加载 dotenv 文件:
import Hummingbird
import Logging
func buildApplication(
configuration: HBApplicationConfiguration
) async throws -> some HBApplicationProtocol {
var logger = Logger(label: "hummingbird-logger")
logger.logLevel = .trace
let env = HBEnvironment.shared
// let env = try await HBEnvironment.dotEnv()
let logLevel = env.get("LOG_LEVEL")
if let logLevel, let logLevel = Logger.Level(rawValue: logLevel) {
logger.logLevel = logLevel
}
let router = HBRouter()
router.get("/") { _, _ in
return "Hello"
}
let app = HBApplication(
router: router,
configuration: configuration,
logger: logger
)
return app
}
If you run the project from Xcode, make sure you set a custom working directory, otherwise the framework won't be able to locate your dotenv file.
如果您从 Xcode 运行项目,请确保设置自定义工作目录,否则框架将无法找到您的 dotenv 文件。
What's next? 下一步是什么?
Environment variables are crucial for modifying application behavior without code changes, offering flexibility across environments. They're commonly used for feature flags, secrets, and other configuration purposes. In our next article we will discover how to store secrets and API credentials in a secure way.
环境变量对于在不更改代码的情况下修改应用程序行为至关重要,从而提供跨环境的灵活性。它们通常用于功能标志、秘密和其他配置目的。在下一篇文章中,我们将了解如何以安全的方式存储机密和 API 凭证。