Modern computers are incredibly fast, and getting faster all the time. However, computers also have some significant constraints: they only natively understand a limited set of commands, and must be told exactly what to do.
现代计算机的速度非常快,而且速度一直在加快。然而,计算机也有一些重要的限制:它们本身只能理解一组有限的命令,并且必须确切地告诉它们该做什么。
A computer program (also commonly called an application) is a set of instructions that the computer can perform in order to perform some task. The process of creating a program is called programming. Programmers typically create programs by producing source code (commonly shortened to code), which is a list of commands typed into one or more text files.
计算机程序(通常也称为应用程序)是计算机可以执行的一组指令,以便执行某些任务。创建程序的过程称为编程。程序员通常通过生成源代码(通常缩写为代码)来创建程序,源代码是键入到一个或多个文本文件中的命令列表。
The collection of physical computer parts that make up a computer and execute programs is called the hardware. When a computer program is loaded into memory and the hardware sequentially executes each instruction, this is called running or executing the program.
构成计算机并执行程序的物理计算机部件的集合称为硬件。当计算机程序被加载到内存中并且硬件按顺序执行每条指令时,这称为运行或执行程序。
Machine Language 机器语言
A computer’s CPU is incapable of speaking C++. The limited set of instructions that a CPU can understand directly is called machine code (or machine language or an instruction set).
计算机的 CPU 无法使用 C++。CPU 可以直接理解的有限指令集称为机器代码(或机器语言或指令集)。
Here is a sample machine language instruction: 10110000 01100001
下面是一个示例机器语言指令: 10110000 01100001
Back when computers were first invented, programmers had to write programs directly in machine language, which was a very difficult and time-consuming thing to do.
当计算机刚被发明时,程序员必须直接用机器语言编写程序,这是一件非常困难和耗时的事情。
How these instructions are organized is beyond the scope of this introduction, but it is interesting to note two things. First, each instruction is composed of a sequence of 1s and 0s. Each individual 0 or 1 is called a binary digit, or bit for short. The number of bits that make up a single command varies -- for example, some CPUs process instructions that are always 32 bits long, whereas some other CPUs (such as the x86/x64 family, which you may be using) have instructions that can be a variable length.
这些说明的组织方式超出了本介绍的范围,但需要注意两件事。首先,每条指令都由 1 和 0 的序列组成。每个单独的 0 或 1 称为二进制数字,或简称位。组成单个命令的位数各不相同,例如,某些 CPU 处理的指令长度始终为 32 位,而其他一些 CPU(如您可能正在使用的 x86/x64 系列)的指令长度可以是可变的。
Second, each set of binary digits is interpreted by the CPU into a command to do a very specific job, such as compare these two numbers, or put this number in that memory location. However, because different CPUs have different instruction sets, instructions that were written for one CPU type could not be used on a CPU that didn’t share the same instruction set. This meant programs generally weren’t portable (usable without major rework) to different types of system, and had to be written all over again.
其次,每组二进制数字都被 CPU 解释为一个命令来执行非常具体的工作,例如比较这两个数字,或将这个数字放在该内存位置。但是,由于不同的 CPU 具有不同的指令集,因此为一种 CPU 类型编写的指令不能在不共享相同指令集的 CPU 上使用。这意味着程序通常不能移植到不同类型的系统(无需重大返工即可使用),并且必须重新编写。
Assembly Language 汇编语言
Because machine language is so hard for humans to read and understand, assembly language was invented. In an assembly language, each instruction is identified by a short abbreviation (rather than a set of bits), and names and other numbers can be used.
由于机器语言对人类来说很难阅读和理解,因此发明了汇编语言。在汇编语言中,每条指令都由一个简短的缩写(而不是一组位)标识,并且可以使用名称和其他数字。
Here is the same instruction as above in assembly language: mov al, 061h
以下是与上述汇编语言相同的说明: mov al, 061h
This makes assembly much easier to read and write than machine language. However, the CPU can not understand assembly language directly. Instead, the assembly program must be translated into machine language before it can be executed by the computer. This is done by using a program called an assembler. Programs written in assembly languages tend to be very fast, and assembly is still used today when speed is critical.
这使得汇编比机器语言更容易读写。但是,CPU 无法直接理解汇编语言。相反,汇编程序必须先翻译成机器语言,然后才能由计算机执行。这是通过使用称为汇编程序的程序完成的。用汇编语言编写的程序往往非常快,当速度至关重要时,汇编今天仍在使用。
However, assembly still has some downsides. First, assembly languages still require a lot of instructions to do even simple tasks. While the individual instructions themselves are somewhat human readable, understanding what an entire program is doing can be challenging (it’s a bit like trying to understand a sentence by looking at each letter individually). Second, assembly language still isn’t very portable -- a program written in assembly for one CPU will likely not work on hardware that uses a different instruction set, and would have to be rewritten or extensively modified.
但是,组装仍然存在一些缺点。首先,汇编语言仍然需要大量的指令来执行简单的任务。虽然单个指令本身在某种程度上是人类可读的,但理解整个程序正在做什么可能具有挑战性(这有点像试图通过单独查看每个字母来理解一个句子)。其次,汇编语言仍然不是很可移植——用汇编语言为一个CPU编写的程序可能无法在使用不同指令集的硬件上运行,并且必须重写或大量修改。
High-level Languages 高级语言
To address the readability and portability concerns, new programming languages such as C, C++, Pascal (and later, languages such as Java, Javascript, and Perl) were developed. These languages are called high level languages, as they are designed to allow the programmer to write programs without having to be as concerned about what kind of computer the program will be run on.
为了解决可读性和可移植性问题,开发了新的编程语言,如C,C++,Pascal(以及后来的语言,如Java,Javascript和Perl)。这些语言被称为高级语言,因为它们旨在允许程序员编写程序,而不必关心程序将在哪种计算机上运行。
Here is the same instruction as above in C/C++: a = 97;
以下是上面 C/C++ 中的相同说明: a = 97;
Much like assembly programs, programs written in high level languages must be translated into a format the computer can understand before they can be run. There are two primary ways this is done: compiling and interpreting.
与汇编程序非常相似,用高级语言编写的程序必须翻译成计算机可以理解的格式,然后才能运行。有两种主要方式:编译和解释。
A compiler is a program (or collection of programs) that reads source code (typically written in a high-level language) and translates it into some other language (typically a low-level language, such as assembly or machine language, etc…). Most often, these low-level language files are then combined into an executable file (containing machine language instructions) that can be run or distributed to others. Notably, running the executable file does not require the compiler to be installed.
编译器是一个程序(或程序集合),它读取源代码(通常用高级语言编写)并将其翻译成其他语言(通常是低级语言,如汇编语言或机器语言等)。大多数情况下,这些低级语言文件被合并成一个可执行文件(包含机器语言指令),可以运行或分发给其他人。值得注意的是,运行可执行文件不需要安装编译器。
In the beginning, compilers were primitive and produced slow, unoptimized code. However, over the years, compilers have become very good at producing fast, optimized code, and in some cases can do a better job than humans can in assembly language!
一开始,编译器是原始的,生成缓慢的、未优化的代码。然而,多年来,编译器已经变得非常擅长生成快速、优化的代码,在某些情况下,编译器在汇编语言方面可以比人类做得更好!
Here is a simplified representation of the compiling process:
以下是编译过程的简化表示:
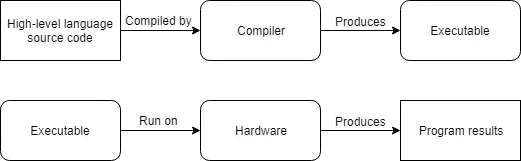
Since C++ programs are generally compiled, we’ll explore C++ compilers in more detail shortly.
由于 C++ 程序通常是编译的,因此我们稍后将更详细地探讨 C++ 编译器。
An interpreter is a program that directly executes the instructions in the source code without requiring them to be compiled into an executable first. Interpreters tend to be more flexible than compilers, but are less efficient when running programs because the interpreting process needs to be done every time the program is run. This also means the interpreter must be installed on every machine where an interpreted program will be run.
解释器是一种程序,它直接执行源代码中的指令,而不需要先将它们编译成可执行文件。解释器往往比编译器更灵活,但在运行程序时效率较低,因为每次运行程序时都需要完成解释过程。这也意味着解释器必须安装在运行解释程序的每台机器上。
Here is a simplified representation of the interpretation process:
以下是解释过程的简化表示:

Optional reading 可选阅读
A good comparison of the advantages of compilers vs interpreters can be found here.
编译器与解释器的优势可以在这里找到一个很好的比较。
Another advantage of compiled programs is that distributing a compiled program does not require distributing the source code. In a non-open-source environment, this is important for IP protection purposes.
编译程序的另一个优点是分发编译程序不需要分发源代码。在非开源环境中,这对于 IP 保护目的非常重要。
Most languages can be compiled or interpreted. Traditionally languages like C, C++, and Pascal are compiled, whereas “scripting” languages like Perl and Javascript tend to be interpreted. Some languages, like Java, use a mix of the two.
大多数语言都可以被编译或解释。传统上,像C,C++和Pascal这样的语言是编译的,而像Perl和Javascript这样的“脚本”语言往往是被解释的。某些语言(如 Java)混合使用两者。
High level languages have many desirable properties.
高级语言具有许多理想的属性。
First, high level languages are much easier to read and write because the commands are closer to natural language that we use every day. Second, high level languages require fewer instructions to perform the same task as lower level languages, making programs more concise and easier to understand. In C++ you can do something like a = b * 2 + 5;
in one line. In assembly language, this would take 5 or 6 different instructions.
首先,高级语言更容易阅读和编写,因为这些命令更接近我们每天使用的自然语言。其次,与低级语言相比,高级语言需要更少的指令来执行相同的任务,使程序更简洁、更易于理解。在 C++ 中,您可以在一行中执行类似 a = b * 2 + 5;
操作。在汇编语言中,这需要 5 或 6 条不同的指令。
Third, programs can be compiled (or interpreted) for many different systems, and you don’t have to change the program to run on different CPUs (you just recompile for that CPU). As an example:
第三,程序可以针对许多不同的系统进行编译(或解释),并且您不必更改程序以在不同的 CPU 上运行(您只需针对该 CPU 重新编译即可)。举个例子:
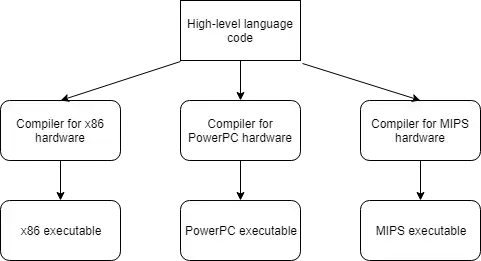
There are two general exceptions to portability.
可移植性有两个一般例外。
The first is that many operating systems, such as Microsoft Windows, contain platform-specific capabilities that you can use in your code. These can make it much easier to write a program for a specific operating system, but at the expense of portability. In these tutorials, we will avoid any platform specific code.
首先,许多操作系统(如 Microsoft Windows)都包含可在代码中使用的特定于平台的功能。这些可以使为特定操作系统编写程序变得更加容易,但以牺牲可移植性为代价。在这些教程中,我们将避免使用任何特定于平台的代码。
The second is that some compilers also support compiler-specific extensions -- if you use these, your programs won’t be able to be compiled by other compilers that don’t support the same extensions without modification. We’ll talk more about these later, once you’ve installed a compiler.
其次,一些编译器还支持特定于编译器的扩展——如果你使用这些扩展,你的程序将无法被其他不支持相同扩展的编译器编译,而这些编译器不经过修改。安装编译器后,我们将在稍后详细讨论这些内容。
Rules, Best practices, and warnings
规则、最佳做法和警告
As we proceed through these tutorials, we’ll highlight many important points under the following three categories:
在继续学习这些教程时,我们将重点介绍以下三个类别下的许多要点:
Rule 统治
Rules are instructions that you must do, as required by the language. Failure to abide by a rule will generally result in your program not working.
规则是您必须按照语言的要求执行的指令。不遵守规则通常会导致您的程序无法运行。
Best practice 最佳实践
Best practices are things that you should do, because that way of doing things is either conventional (idiomatic) or recommended. That is, either everybody does it that way (and if you do otherwise, you’ll be doing something people don’t expect), or it is superior to the alternatives.
最佳实践是你应该做的事情,因为这种做事方式要么是传统的(惯用的),要么是推荐的。也就是说,要么每个人都这样做(如果你不这样做,你就会做一些人们意想不到的事情),要么它优于替代方案。
Warning 警告
Warnings are things that you should not do, because they will generally lead to unexpected results.
警告是你不应该做的事情,因为它们通常会导致意想不到的结果。
2024 年 5 月 2 日 下午 12:32
Either me or someone will make a language that can program everything from making a website to making games and everything
无论是我还是某人,都会制作一种语言,可以对从制作网站到制作游戏等一切内容进行编程
else that is also fast, secure and efficient. What i am picturing in my mind right now is the perfect programming language. it has to happen, surely. this is my goal right now. And no way, it is impossible to do.
否则,这也是快速、安全和高效的。我现在在脑海中想象的是完美的编程语言。当然,它必须发生。这是我现在的目标。没办法,这是不可能的。
2024 年 5 月 3 日 凌晨 4 点 09 分
it is impossible. every language has its pros and cons and sacrifices simplicity/complexity/syntax/garbage collection etc etc for other things. In computer science there will never be a "one fit all". There won't be a perfect memory (hdd, ssd, ram, caches serve different purposes), a perfect language, a perfect CPU etc.
这是不可能的。每种语言都有其优点和缺点,并牺牲了简单性/复杂性/语法/垃圾收集等。在计算机科学中,永远不会有“一刀切”。不会有完美的内存(硬盘、固态硬盘、内存、缓存有不同的用途)、完美的语言、完美的 CPU 等。
it is not impossimble at all python is being used to make apps websites but it has its disadvantages. A language for all is possible for example there could be libraries that tap into low level and libraries for high level like java. Is it worth it f no but is it possible yes
Exactly what I am saying, since python is designed for simplicity, it can't be used for low level things. It's an interpretated language, thus it will never be faster than C, thus not used in low level programming. Every language is designed with a certain purpose, and while you can use them for other things as well, it won't be the better choice and thus it won't be used for that matter.
Summer Plan, learn C++/C in 3 months. Let's get it!
When it says that the executable file can be run or distributed to others, is that just saying that once it is compiled into an executable file, it can be executed on different hardware?
An executable will be runable on any system that is compatible with the target architecture and OS that the executable was compiled for. For example, a modern executable compiled for Win 64 should be runnable on Win 10/11 and any x64 64-bit CPU (e.g. AMD or Intel).
let's see how far I got. I'll be back in few years
Wish me luck y’all. I’m starting this journey and I plan on seeing through to the end!
how did you get so far ?
Did you learn c++?
Did you get a job?
Did you make your own programms?
It’s been two days sir! But thanks!
Haha here I was excited because reading HaosKid's question I just assumed your comment must have been many years old.
Well, I am diving in too. A new goal in life with a very ripe age. Wish me luck.
Good luck to you julio! I hope you achieve what you set out to do!
I'm need to learn this to understand better my ros code.
Starting over again. Wish me luck.
Me too brother. Good luck to you.
I was expecting homework!
wish me luck chat
here we go again